How to Use React in HubSpot CMS to Create Customized Web Experiences
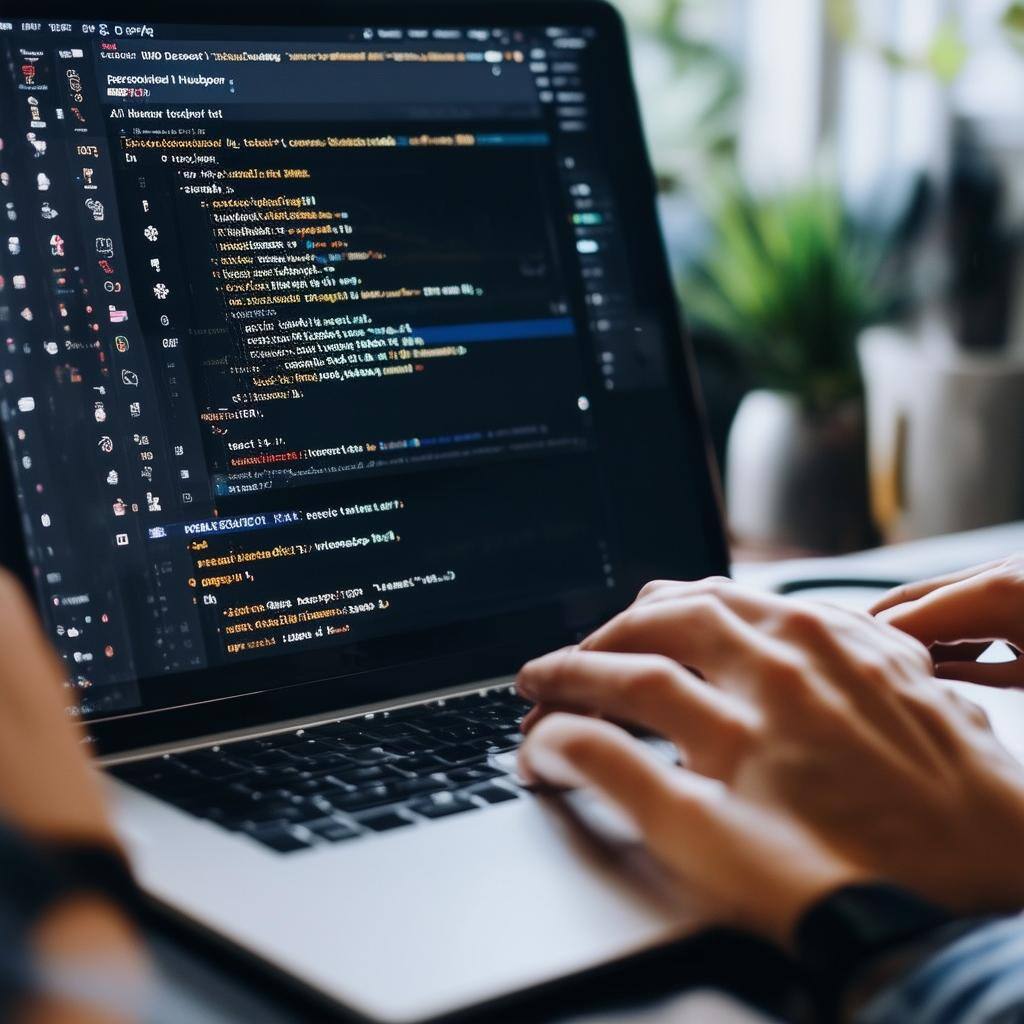
In this post, we will explore how React, one of the most popular JavaScript libraries, integrates with and is used within HubSpot CMS to develop more dynamic and customized web experiences. We’ll divide the content into two sections: an accessible explanation for beginners and a more technical one for developers who want to implement advanced solutions in HubSpot CMS.
Part 1: Introduction to React in HubSpot CMS (For Beginners)
What is React and Why Use It in HubSpot?
React is a JavaScript library developed by Facebook that simplifies the creation of interactive user interfaces (UI) and reusable components. One of React’s main advantages is that it allows you to build dynamic, responsive applications that efficiently update with data changes.
HubSpot CMS is a powerful platform that enables marketing and development teams to create and manage websites. By using React in HubSpot, you can add advanced functionalities and personalize user experiences, which can be very beneficial for companies seeking to stand out and deliver interactive, relevant content.
How is React Integrated into HubSpot CMS?
Integrating React into HubSpot CMS is relatively straightforward but requires a basic understanding of HTML, CSS, and JavaScript. The integration is typically done in two ways:
- React Modules in HubSpot: HubSpot’s modules allow developers to add custom components to the CMS. These modules can contain React and integrate into HubSpot pages like any other module.
- Single Page Applications (SPA): You can build single-page applications using React that interact directly with HubSpot’s API to retrieve dynamic data and display personalized content.
Benefits of Using React in HubSpot
- Advanced Interactivity: React makes it easy to create interactive elements like dynamic forms, updatable charts, and filterable lists.
- Personalized User Experience: With React, you can create personalized experiences based on user behavior and data stored in HubSpot.
- Optimized Performance: React updates only the parts of the page that change, improving performance and load speed.
Simple Example: A Counter with React
To get started, let’s create a simple counter using React within HubSpot.
-
Create a Custom Module: Go to the design section in HubSpot and create a new custom module. Inside the module, include a container for React:
<div id="react-counter"></div> -
Include React in the Module: Add React and ReactDOM scripts to your module using these links:
<script src="https://unpkg.com/react@17/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@17/umd/react-dom.development.js"></script> -
Add the Counter Script: Next, add this script to create a React component that increments a counter each time a button is clicked.
javascript<script type="text/javascript">
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h1>Counter: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
ReactDOM.render(<Counter />, document.getElementById('react-counter'));
</script>
This simple component displays a button that increments a counter when clicked. It’s a basic demonstration of how React can be integrated into HubSpot to add interactive functionality.
Part 2: Technical Implementation of React in HubSpot (For Advanced Developers)
Creating Custom Modules with React and HubL
HubSpot uses HubL (HubSpot Markup Language), its own templating language, to render dynamic content within the CMS. When combined with React, you can create highly functional, reusable custom modules.
Step 1: Project Setup To work with React in HubSpot, it’s recommended to use HubSpot’s local development environment (HubSpot CLI). This allows for more efficient development and smoother integration with the platform.
- Initialize the Project: Create a React project using
create-react-app
. - Configure the Build Folder: Set the
dist
folder as the build destination for static files. - Compile the Project: Configure webpack to bundle your React project into a JavaScript file that can be imported into a HubSpot module. Ensure the final file compiles to an ES5-compatible format to support all browsers supported by HubSpot.
Step 2: Create the Module in HubSpot
-
Module Structure: Create a custom module in HubSpot with a basic HTML file that serves as the container for your React component. Add module variables to allow customization from HubSpot’s UI.
html<div id="my-react-component">
</div> -
Import the Compiled Script: Add the compiled React script to the module, ensuring it loads after the container.
<script src=""></script>
-
Render the React Component: In your React file, ensure the component mounts in the correct container using
ReactDOM.render()
.ReactDOM.render(<MyComponent />, document.getElementById('my-react-component'));
Step 3: Connecting React with HubSpot’s CRM One of HubSpot’s most powerful features is its CRM. By using HubSpot’s API within React, you can create personalized experiences based on user information.
-
Authentication: You’ll need an API token to authenticate requests to the HubSpot API.
-
API Requests: Use
fetch
oraxios
to make requests to HubSpot’s CRM API from your React component.axios.get('https://api.hubspot.com/contacts/v1/lists/all/contacts/all', {
headers: {
Authorization: `Bearer YOUR_ACCESS_TOKEN`
}
}).then(response => {
console.log(response.data);
}); -
Update Component State with CRM Data: Use the data from the API to update your component’s state and render dynamic content.
componentDidMount() {
axios.get('https://api.hubspot.com/contacts/v1/lists/all/contacts/all', {
headers: {
Authorization: `Bearer YOUR_ACCESS_TOKEN`
}
}).then(response => {
this.setState({ contacts: response.data.contacts });
});
}
Best Practices and Tips
- Load Optimization: Use lazy loading to load React components only when needed, reducing initial page load time.
- Modular Development: Divide your application into small, reusable components that can be managed and updated independently.
- Security: Never expose API tokens or sensitive information in your source code. Use environment variables and other techniques to protect your data.
Conclusion
Using React in HubSpot CMS opens up a world of possibilities for developers looking to create dynamic and personalized web experiences. From interactive modules to complete applications, React allows you to maximize HubSpot CMS’s capabilities, enhancing user experience and simplifying CRM integration.
For those just starting out, implementing basic components is a great starting point. More experienced developers can use advanced techniques to create integrated applications that fully leverage HubSpot’s platform. In any case, combining React with HubSpot provides a significant opportunity to take your web projects to the next level.